PowerShell - Script-A-Day for 1 week
- Sydnie Barnes
- Jun 8, 2024
- 4 min read
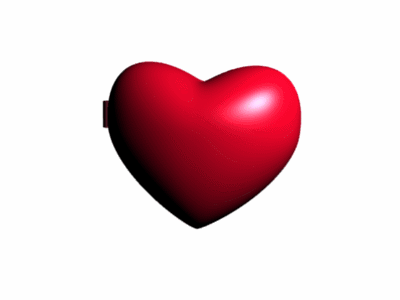
I love working in PowerShell in a corporate environment. It's an automation-minded person's best friend while working in a role that dictates a lot of repetitive tasks. I've decided that I want to come up with and try to write a little script daily for one work week. We'll see how it goes!
Day 1-2: Write a script to add or remove common AD groups from KNOWN AD users
Nothing too fancy, but something that can be configured to add / remove groups and check if a user exists in AD.
# Import Modules
Import-Module ActiveDirectory
# Groups - can be adjusted
$group1 = "changeme"
$group2 = "changeme"
$group3 = "changeme"
# Reset Variable
$userValid = $false
# Checks to see if the user exists in AD
while (-not $userValid) {
# Assign a user to action
$user = Read-Host "Please enter the firstname.lastname of the user to action."
Write-Host "User set to $user - searching AD for user..."
if (Get-ADUser -Filter "samaccountname -eq '$user'") {
Write-Host "User found!" -ForegroundColor Green
$userValid = $true
} else {
Write-Host "User not found. Please try again." -ForegroundColor Yellow
$userValid = $false
}
}
Write-Host "Group adjuster!" -ForegroundColor Cyan
$groupAction = Read-Host "Would you like to add (A) or remove (R) groups? Press (Q) to quit."
switch ($groupAction) {
"A" { # Adds groups 1 and 2 and removes group 3.
Write-Host "Adding $group1 and $group2..."
Add-ADGroupMember $group1 $user
Add-ADGroupMember $group2 $user
Write-Host "Done!" -ForegroundColor Green
Write-Host "Removing $group3..."
Remove-ADGroupMember $group3 $user -Confirm:$false
Write-Host "Done!" -ForegroundColor Green
Write-Host "Groups have been adjusted - here are $user's new groups"
Get-ADUser $user -Properties MemberOf | Select-Object -ExpandProperty MemberOf | Get-ADGroup | Select-Object name | Sort-Object name | Format-Table
Write-Host "User groups have been displayed. This screen will stay up for 30 seconds unless you close it before then"
Start-Sleep -s 30
}
"R" { # Removes Dynamics groups and adds an E3 license.
Write-Host "Removing $group1 and $group2..."
Remove-ADGroupMember $group1 $user -Confirm:$false
Remove-ADGroupMember $group2 $user -Confirm:$false
Write-Host "Done!" -ForegroundColor Green
Write-Host "Adding $group3..."
Add-ADGroupMember $group3 $user
Write-Host "Done!"
Write-Host "Groups have been adjusted - here are $user's new groups"
Get-ADUser $user -Properties MemberOf | Select-Object -ExpandProperty MemberOf | Get-ADGroup | Select-Object name | Sort-Object name | Format-Table
Write-Host "User groups have been displayed. This screen will stay up for 30 seconds unless you close it before then"
Start-Sleep -s 30
}
"Q" {
Break
}
Default {
Write-Host "Please make a valid selection."
}
}
Day 3: Writing a script to automatically sort my photos by date
I love photography. I love shooting photos equally as much as I hate sorting them! My old process involved copying files & raws and going through them manually then deleting them from the camera. This script takes folders from a folder and divides them into folders by date. This makes sorting just about any folder much easier.
# Set path - can be changed
$sourcePath = ""
# Get all files in the directory
Get-ChildItem $sourcePath | ForEach-Object {
$file = $_.FullName
$date = Get-Date ($_.LastWriteTime)
$year = $date.Year
$month = $date.Month
$day = $date.Day
$MonthPath = "$sourcePath\$year-$month-$day"
# Create the folder if it doesn't exist
if (!(Test-Path -Path $MonthPath)) {
New-Item -ItemType directory -Path $MonthPath | Out-Null
}
# Move the file to the appropriate folder
Move-Item $file $MonthPath | Out-Null
}
Write-Host "Files have been sorted into folders."
Day 4: Get a list of installed programs and export to a .csv
# Assign a path to export the CSV
$path = "changeme"
# Pulls the computer name that the script is run on
$computerName = $env:computername
Get-Package -ProviderName Programs | Select-Object Name,Version | Sort-Object Name | Export-Csv "$path\$computerName-InstalledPrograms.csv" -NoTypeInformation
Day 5: Back up user data from a failed (or failing) hard drive
Fairly often in my work, we'll need to recover the configurations of PCs which don't boot to windows. Here's a script I wrote to recover data, including any browser profiles (because these same users also seldom sign into browsers). Ideally, one plugs in an external data source to copy the data to.
# Sets a destination path to copy user data to
$destinationPath = "D:\"
# Gets a list of users on the HD
$users = Get-LocalUser | Where-Object Enabled
Write-Host "Users found!"
Write-Output $users
# Copy any user documents to the destination path
Write-Host "Begining data export to $destinationPath"
foreach ($user in $users) {
Write-Host "Beginning copy of $user data..."
$userPath = "$destinationPath\$user"
# Creates a new folder for the user data in the destination path
if (!(Test-Path -Path $userPath)) {
New-Item -ItemType directory -Path $userPath | Out-Null
}
Set-Location "C:\Users\$user"
Copy-Item .\Downloads -Destination $userPath -Recurse
Write-Host "$user Downloads copied!"
Copy-Item .\Desktop $userPath -Recurse
Write-Host "$user Desktop copied!"
Copy-Item .\Documents $userPath -Recurse
Write-Host "$user Documents copied!"
Copy-Item .\Pictures $userPath -Recurse
Write-Host "$user Pictures copied!"
Copy-Item .\Videos $userPath -Recurse
Write-Host "$user Videos copied!"
Copy-Item -Path '.\AppData\Local\Google\Chrome\User Data' -Destination $destinationPath\ChromeUserData
Copy-Item -Path '.\AppData\Local\Microsoft\Edge\User Data' -Destination $destinationPath\EdgeUserData
Write-Host "$user Chrome & Edge user data copied!"
# Generate a list of installed programs and exports as a csv
$computerName = $env:computername
Get-Package -ProviderName Programs | Select-Object Name,Version | Sort-Object Name | Export-Csv "$userPath\$computerName-InstalledPrograms.csv" -NoTypeInformation
Write-Host "$user Programs list exported!"
Write-Host "Data has been copied successfully to $userPath"
}
Comments